CSCI 112
Lab 5 - Looping, looping, and even more of looping...
Objectives:
- Design and implement an algorithm using nested
loops.
- Learn to use both while and for loops.
Readings:
- Read Chapter 5 in the Hanly/Koffman text.
Deliverables (DUE BY
THE MIDNIGHT ON THE DAY OF LAB!):
- Submittal of your algorithm and two
files: lab5.c
and Makefile.
- The algorithm needs to be provided in the form of the flowchart, which was introduced
during the lecture on Top-Down Design with
Functions. You can draw the symbols by hand, or use any type of
software with diagram-drawing capabilities (e.g.
MS Visio, or some free
ones: OpenOffice.org Draw, Dia). Your program’s
code needs to match the algorithm you submitted.
TO DO (for today's
lab)
- Design a program in C which
prints a series like the following for an input of an odd number, which is
smaller or equal to 9. The pattern below represents the most extreme case
that can be printed (i.e. the user entered 9 as the input).
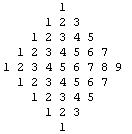
- As you
can observe from the series presented above, the user is expected to
provide an input which is odd. Make sure your user puts in an odd number
less than 10 and force her to input until she inputs an odd number.
- Use
both while and for
loops, and implement the solution using nested loops.
- For
example, a typical session may look in the following way:
Enter an odd number less than equal to
9: 6
You have inputted an even number.
Please try again!
Enter an odd number less than equal to
9: 11
You have inputted a number greater
than 9. Please try again!
Enter an odd number less than equal to
9: 9
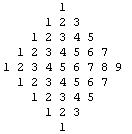
- Note: You will loose a
substantial percentage of points if you use only one type of loop
or your output does not follow the pattern as
shown above.
LAB 5 ENRICHMENT
- Rewrite your code using do-while loop to take the input
from the user.
- Rewrite
your code in such way that you do not use neither "<=" nor ">="
relational operators (you are allowed to use "<"
or ">" or other operators)
- Can
you generate the same output, when using only decremented
counters (e.g. i = i - 1 )? How about
using only prefix decrements (e.g. --i)?