CSCI 112
Lab 7 - Enumerated Type and Function Parameters
Objectives:
- Design and implement a program to solve the problem
described below.
- Practice use of loops, switch statements, enumerated
types and passing function parameters.
Readings:
- Read about Enumerated Types in the Hanly/Koffman text
(pp. 407-414 in 7th edition, pp. 340-346 in 5th edition, people who have 6th
edition - please, search for relevant section using the index at the end).
- BTW, to the guy in our class
who borrowed the 6th edition from me over 3 weeks ago and never returned it
(paraphrasing from the movie Taken) - "I don't have money. But what I do
have are a very particular set of skills; skills I have acquired over a very
long career. Skills that make me a nightmare for people like you. If you
bring my book back within a week, that'll be the end of it. I will not
punish you, I will not pursue you. But if you don't, I will look for you, I
will find you, and I will get my book back." THIS IS A JOKE, BUT I DO
NEED MY BOOK BACK!!!!
Deliverables: (DUE: Midnight on March 9th,
2013)
- Submittal of the following files:
- Makefile
- proto.h
- lab7.c
- get_problem.c
- deg_to_rad.c
- evaluate_sin.c
- evaluate_cos.c
- evaluate_tan.c
TO DO (for this
lab)
- Design and Write a program that asks the user to
enter the number of a method, that represents a trigonometric function,
and then calculates the values for the chosen function for every 15
degrees, starting from 0 degree until the 90 degree is reached (e.g. sin(45.00) = 0.71).
Please
choose an option from the menu below by entering a number from 0 to 3 (enter 3
to exit this program).
(0)
Sine
(1)
Cosine
(2)
Tangent
(3)
QUIT
- The last option (QUIT) lets the user immediately
exit the program.
- Note that trigonometric functions like sin(x) or cos(x), only accept the
parameter in the form of a radian
value. The relationship between degree and radian is: radian = π * degree / 180.
- Because tan(90.00) is an infinite number, you
need to make sure that in place of a value, that is originally returned by
the tan(90.00) function call, you display UNDEFINED in your program.
Otherwise, your application will generate an incorrect value.
- You should use a counter-controlled
loop (any kind) to print out lines with a value of a chosen trigonometric
function for every 15 degrees (e.g. in the first line: tan(0.00) = 0.00, in the second
line: tan(15.00) = 0.27,
etc.).
- You are expected to define and use two global
constants: PI
as 3.14159 value, and LOOP_LIMIT as 90.00 degree. The constants
need to be placed in proto.h file.
- A sentinel-controlled
loop is recommended to check for a QUIT option in your menu.
- Define enumerated type in proto.h to reflect your
four-choices menu (i.e. Sine,
Cosine, Tangent, QUIT),
and use it in the sentinel-controlled
loop. Switch-type statement may also prove itself to be helpful. :)
- Make sure to place a flag in proto.h for conditional
compilation. Check out my
slides, if you forgot how to write one.
- Here is a short characterization of the functions you
need to write in separate files:
- get_problem.c
–
Displays menu with trigonometric methods and lets the user make the
choice.
- deg_to_rad.c
–
Transforms the degree to the appropriate radian value.
- evaluate_sin.c,
evaluate_cos.c, evaluate_tan.c – Print out single lines with trigonometric
functions for each degree passed as the input parameter. These functions should NOT contain
any loops in their bodies.
- Make sure to comment your code (e.g. functions)!
- Here is an example of display your program should
generate (if you choose every method one time, #'s represent your
calculated data):
Please
choose an option from the menu below by entering a number from 0 to 3 (enter 3
to exit this program).
(0)
Sine
(1)
Cosine
(2)
Tangent
(3)
QUIT
The
value that was entered is: 0
sin(0.00)
= #.##
sin(15.00)
= #.##
sin(30.00)
= #.##
sin(45.00)
= #.##
sin(60.00)
= #.##
sin(75.00)
= #.##
sin(90.00)
= #.##
Please
choose an option from the menu below by entering a number from 0 to 3 (enter 3
to exit this program).
(0)
Sine
(1)
Cosine
(2)
Tangent
(3)
QUIT
The
value that was entered is: 1
cos(0.00)
= #.##
cos(15.00)
= #.##
cos(30.00)
= #.##
cos(45.00)
= #.##
cos(60.00)
= #.##
cos(75.00)
= #.##
cos(90.00)
= #.##
Please
choose an option from the menu below by entering a number from 0 to 3 (enter 3
to exit this program).
(0)
Sine
(1)
Cosine
(2)
Tangent
(3)
QUIT
The
value that was entered is: 2
tan(0.00)
= #.##
tan(15.00)
= #.##
tan(30.00)
= #.##
tan(45.00)
= #.##
tan(60.00)
= #.##
tan(75.00)
= #.##
tan(90.00)
= UNDEFINED
Please
choose an option from the menu below by entering a number from 0 to 3 (enter 3
to exit this program).
(0)
Sine
(1)
Cosine
(2)
Tangent
(3)
QUIT
The
value that was entered is: 3
The
program finished its run. Thank you.
LAB 7 BONUS (5 extra points are available!)
- Extend your code to allow inclusion of a universal stub
for any type of function (to be chosen as option 4 from your menu).
- You will need to build a stub file for TestFunction (the file’s name: TestFunction.c) that will be supplied
after submittal.
- TestFunction Stub:
- Type of function is void.
- Name of function is TestFunction.
- Number of input
parameters is one.
- Type of input parameter
is double.
- Your stub will need to be called properly in your main
program. The value (of type double) it returns should be
properly formatted.
- When we grade your program, we will overwrite your TestFunction.c with our version of TestFunction.c and your program will
then produce an expected output that we will check for. Here is an
example, showing how the updated code could run:
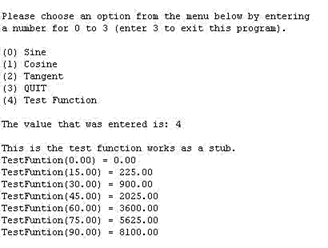
- Note: The values returned by
the test function above are totally dependent of the function, we will
replace your TestFunction.c with, and therefore you do not need to put a lot of
effort into generating the same numbers (i.e. TestFunction‘s output values).